Curious about how you can send an SMS message from Python for free? This article presents a ready-made Python function to send an SMS message. Simply copy-paste the function into your own Python program and voilà, you are all set. The demonstrated Python code to send an SMS, builds on the Textbelt web API. Textbelt allows you to send one SMS for free every day. Great for server monitoring purposes, where you do not expect issue on a regular basis.
Background
Not too long ago I experimented with a different WordPress caching plugin for this site. All seemed to work fine. After about a week, I noticed that all of a sudden web pages no longer loaded properly. I quickly reverted back to the latest backup to limit downtime. Digging a bit deeper, I concluded that the new plugin caused the problem.
Not a big deal. It got me wondering though: “How can I be informed about similar issues”? I decided on writing a quick Python program for monitoring purposes. The Python program runs periodically as a Cron-job on another server. It loads the HTML of the front page, extracts the page title and compares it with the title that I expect. Upon detection of a page title mismatch, I wanted to receive a notification on my phone. Multiple options exist, such as a WhatsApp, a Telegram or an SMS message. I decided on the last one.
That left me with one more question: “How to send an SMS message using Python”? You need a third-party web API for this. I found a really nice one called Textbelt. No need to create an account, simply prepay with PayPal. Best of all, Textbelt allows you to send one SMS from Python for free, each day. For server monitoring you typically do not expect daily issues. Therefore, you could use this approach at no additional cost. After figuring out how to send an SMS from Python, I figured: “Why not share what I learned”. This article teaches you how to send an SMS from Python for free, using the Textbelt web API. Note that I am not affiliated with Textbelt.
What do you need
All Linux desktop distributions come with Python preinstalled. Therefore you just need a Linux based PC with desktop environment, to complete the steps outlined in this article.
Regular readers of this blog know that I prefer PyCharm for Python development. This article is no exception. I recommend installing the free PyCharm Community Edition. That makes it easier to follow along with this article. For details, refer to the article about installing the PyCharm Community Edition as a Flatpak from Flathub.
We’ll be installing a dependency from the Python Package Index (PyPi). To not pollute the global Python environment on your Linux system, we will use a Python virtual environment. This previously published article explains in more detail how and when to use a Python Virtual Environment.
Setup a project in PyCharm
This section covers the setup of our PyCharm project called smsdemo. It includes the creation of the virtual environment and installing the requirements from PyPi.
Create a new project with virtual environment
Start PyCharm and create a new project. On the new project dialog:
- Select the location and name of the project.
- I called the project smsdemo and located it in subdirectory PycharmProjects of my home directory.
- Select the option to create a new virtual environment for the project and specify its location.
- I named it the same as the project and located it in subdirectory venv of my home directory.
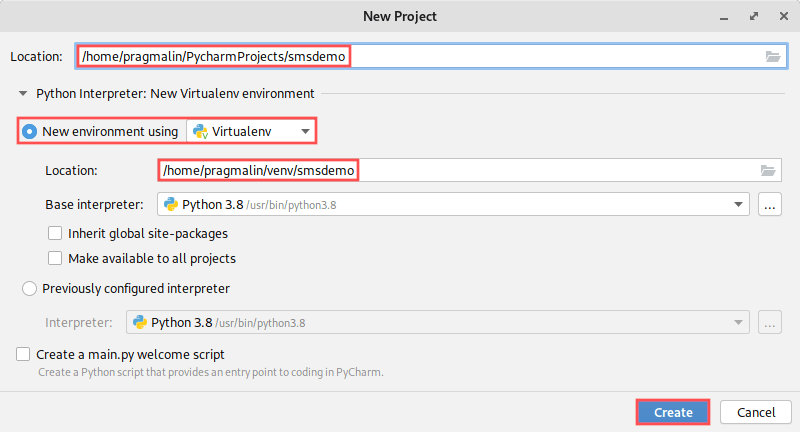
I highly recommend using a separate Python virtual environment for each of your Python projects. You can then install whatever package, even a specific version of a package, just for the project you work on. It won’t affect your system’s Python installation or your other Python projects.
Install packages from PyPi
To send an SMS message from Python, we’ll call the Textbelt web API with the help of the request package. Before we can do so, we need to make sure that the request package is available in our project’s virtual environment. Open up the terminal screen inside the PyCharm IDE and run the command:
pip install "requests"
This installs the requests package from PyPI, if not yet present:
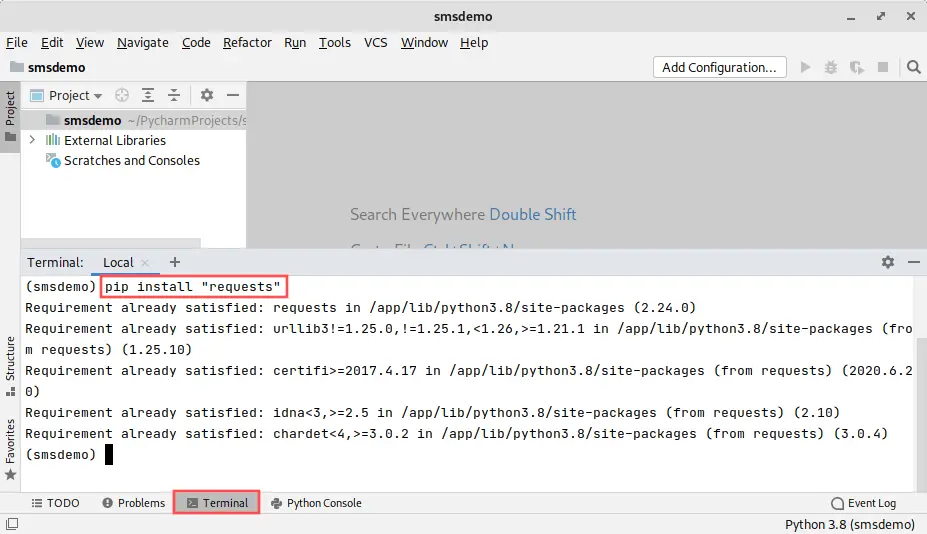
Create the Python program framework
To test that we can send an SMS message from Python, we’ll create an empty Python program as a starting point. Using the PyCharm IDE, create a new Python file in the project, called smsdemo.py
, and enter the following contents:
#!/usr/bin/env python3 import requests def main(): # TODO Enter code here.. pass if __name__ == "__main__": main()
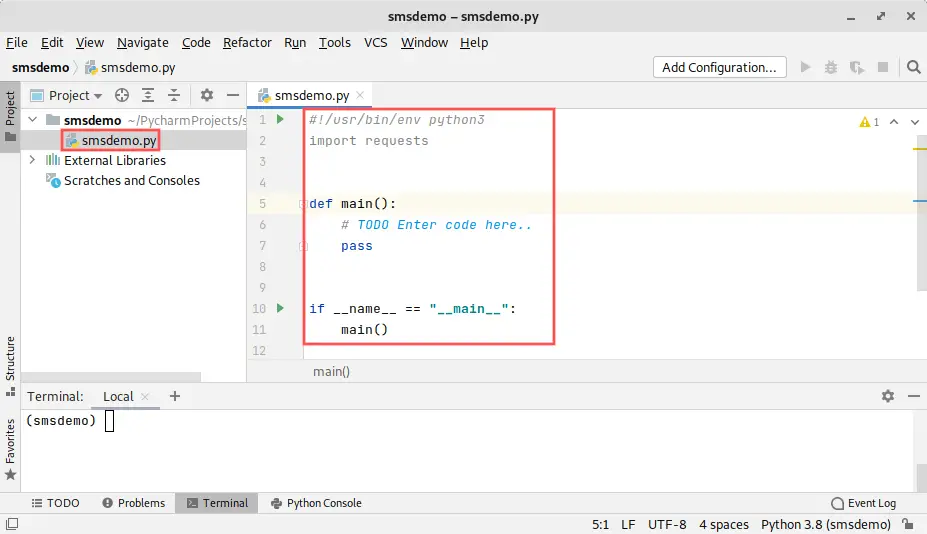
Introduction to the Textbelt API
To send an SMS message from Python, you at least need to know:
- The recipient’s phone number.
- The actual text message, limited to 160 characters.
When using the Textbelt web API, you need one thing extra:
- A so called API key.
Creating a Textbelt API key
To create your individual and unique API key, head over to the Textbelt website and click the Create API key link:
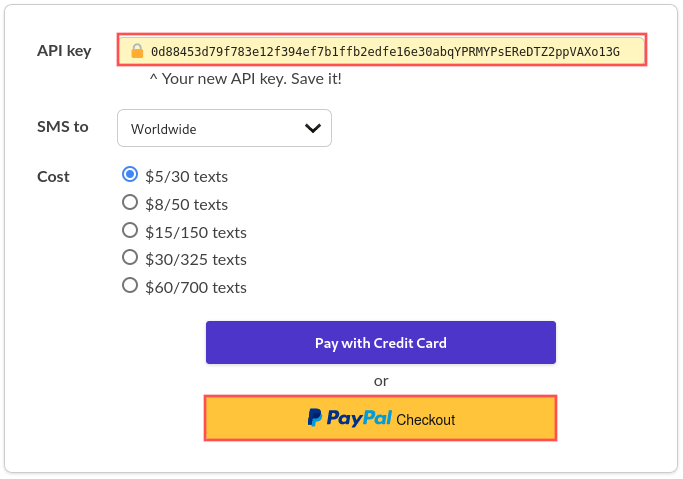
Next, you specify where you intend to send the SMS message to and how many SMS messages you want to purchase. As a final step, you click the PayPal button to purchase that many SMS messages. Once done, you can send that many SMS messages from Python, using the created API key.
But wait, didn’t I say you can send one SMS message for free? Yup, in this case you simply use textbelt as the API key. No need to generate your own API key, if one SMS message per day suffices.
The Textbelt web API
To send an SMS message with Textbelt, you simply post the following key pairs to https://textbelt.com/text
:
phone
: recipient’s phone numbermessage
: text message to sendkey
: api key
In return the Textbelt web API gives you a JSON formatted response. In case all went fine, the response looks like:
{"success": true, "quotaRemaining": 23, "textId": 54793}
When an error was detected, the response depends on the type of error. For the situation where your API key ran out of SMS messages:
{"success": false, "quotaRemaining": 0, "error": "Out of quota"}
If you specified incorrect key pairs, the response would be formatted as:
{"success": false, "error": "Incomplete request"}
Python function to send an SMS message
As you learned in the previous section, to send an SMS message from Python, we need to:
- Post the
phone
,message
andkey
variables to thehttps://textbelt.com/text
URL. - Receive the JSON formatted response.
- Evaluate the boolean
success
value in JSON response.
Refer to the following Python function, which performs exactly these three steps. It includes rudimentary exception handling:
def send_textbelt_sms(phone, msg, apikey): """ Sends an SMS through the Textbelt API. :param phone: Phone number to send the SMS to. :param msg: SMS message. Should not be more than 160 characters. :param apikey: Your textbelt API key. 'textbelt' can be used for free for 1 SMS per day. :returns: True if the SMS could be sent. False otherwise. :rtype: bool """ result = True json_success = False # Attempt to send the SMS through textbelt's API and a requests instance. try: resp = requests.post('https://textbelt.com/text', { 'phone': phone, 'message': msg, 'key': apikey, }) except: result = False # Extract boolean API result if result: try: json_success = resp.json()["success"] except: result = False # Evaluate if the SMS was successfully sent. if result: if not json_success: result = False; # Give the result back to the caller. return result
Go ahead and copy-paste this function into our smsdemo.py
demo program. Place it right underneath function main()
, but before the line:
if __name__ == "__main__":
Send an SMS message for testing purposes
Now that we added the function for sending an SMS message from our Python program, let’s add some example code to test it. Change function main()
to the following:
def main(): """ Send an SMS message for testing purposes. """ phone = '+15558838530' # <-- Enter your own phone number here smsmsg = 'Test message using the Textbelt web API.' apikey = 'textbelt' # <-- Change to your API key, if desired # Attempt to send the SMS message. if send_textbelt_sms(phone, smsmsg, apikey): print('SMS message successfully sent!') else: print('Could not send SMS message.')
Just make sure to change the phone
variable to your actual cellphone number. You can also change the apikey
variable to the API key you created earlier. If you just want to use the free option, you can keep the textbelt
value. Remember that with the textbelt
API key, you can only send one SMS per day.
To run our demo program, select option Run… from the Run program menu in PyCharm. Next, select smsdemo to create a run configuration for the demo program. You can see the output of the demo program at the bottom of the screen, in the Run window:
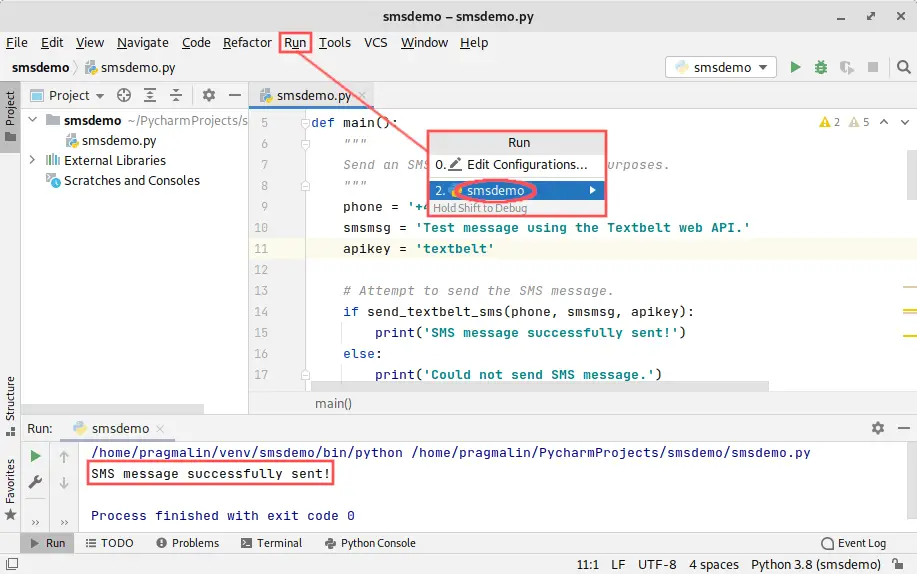
Shortly, you’ll receive the SMS message on your phone:
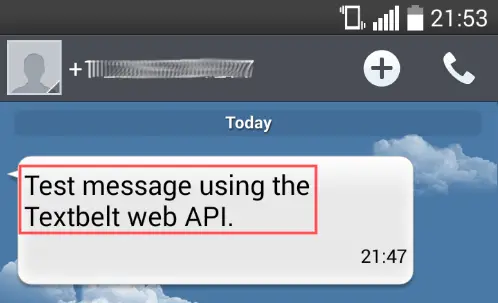
Wrap up
In this article you learned how to send an SMS message from a Python program for free. We used the Textbelt web API for this. To summarize, we completed these steps:
- Created a PyCharm project, including a virtual environment.
- Installed dependencies from PyPi.
- Added the
send_textbelt_sms
Python function for sending an SMS message. - Added test code to function
main()
to see if we can actually send the SMS message.
The Textbelt web API allows you to send one SMS message per day for free. To send more, or to support its developer, you can generate an API key and pre-purchase a few SMS messages on the Textbelt website.
We used Python to send the SMS message. Note though that the Textbelt web API works with different programming languages, such as: Ruby, JavaScript, PHP, Java, Go, C#, etc. For code examples and more information about the web API, visit the Textbelt documentation site.