Noticed that the Raspberry PI 4 runs a bit hotter than previous models? That is the unfortunate side effect of packing an increased amount of processing power in the same small board form factor. This article presents you with code snippets in several programming languages for obtaining the Raspberry PI CPU temperature. You can use these to build functionality into your own software programs for monitoring the Raspberry PI CPU temperature.
Background
After my newly ordered Raspberry PI 4 board arrived in the mail, I dropped everything to take it for a spin. In a matter of minutes my micro SD-card contained the Raspbian Lite image. All ready to setup the Raspberry PI 4 as a headless server. With raised excitement levels, I booted the Raspberry PI 4 for the first time. The first command I ran was of course htop
. After all, I wanted to verify the presence of the full 4 GB of RAM.
To make the board more appealing to look at and to protect it, I placed the Raspberry PI 4 in plastic enclosure. Included in the packaging of the enclosure were a few passive heat-sinks and a fan. When I saw the fan, I though to myself “Wait, why would I need a fan? Surely a fan is superfluous”. So I skipped connecting the fan. After playing around with the Raspberry PI 4 through an SSH session and configuring it such that I can login with an SSH key, I opened the enclosure. Since I didn’t connect the fan, I figured I should probably double-check that the chip doesn’t run too hot. With this in mind, I touched the black Broadcom BCM2711 chip and thought “Ouch”.
Raspberry PI 4 heat generation
As it turns out, all the wonderful new processing power the quad-core 64-bit 1.5 GHz Cortex-A72 CPU offers, comes at a price. The Raspberry PI board has a harder time dissipating CPU chip generated heat. When running the board in the open air, without an enclosure, gluing a few passive heat-sinks on the black chips suffices. However, this might not be enough if you place the board in some sort of housing. In this case active cooling by means of a fan could be required. But how do you know for sure if this is needed?
The design of the Raspberry PI 4 board and the selection of its components allow the CPU to run fine up to 85 degrees Celsius. This means that as long as the CPU temperature stays below this threshold, the chip doesn’t get damaged. Being a programmer at heart, I set out to search for a way to measure the CPU temperature through software. A quick Google search shows you that you can run the vcgencmd measure_temp
command.
Now that the topic of checking the Raspberry PI CPU temperature piqued my interest, my curiosity led me to research a bit further. How can you obtain the CPU temperature from a Python program? And once you figured that out, how can you do the same in other programming languages? This article presents you with code snippets in several programming languages for obtaining the Raspberry PI CPU temperature. For adding this functionality to your own software program, you can easily copy-and-paste these code snippets. For those that want to get to the code snippets right away, you can find them in PragmaticLinux’s CpuTemp GitHub repository.
CPU temperature under Linux
As a result of a little research into measuring CPU temperatures, I learned that the vcgencmd measure_temp
only works under the Raspbian operating system. However, an alternative method exists that also works on other Linux based distributions. For example Debian and Ubuntu. It turns out that the file /sys/class/thermal/thermal_zone0/temp
holds the CPU temperature in degrees Celsius with a factor of 1000. That is to say, you can simply open this file with the Nano editor and look at the CPU temperature:
nano /sys/class/thermal/thermal_zone0/temp
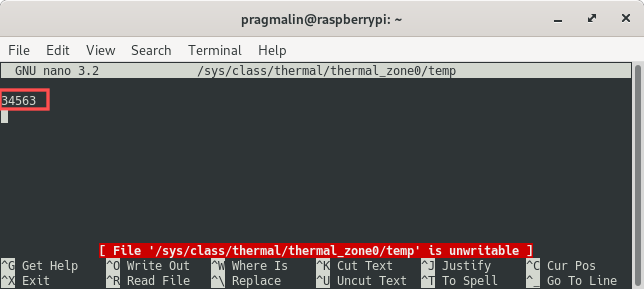
In the screenshot you can see that the my current Raspberry PI CPU temperature is 34.563 degrees Celsius. With this in mind, it becomes clear how to read the CPU temperature in a software program: Start by reading the first line in this file. Next, convert the string to a number. Finally, divide that number by 1000 to get the actual temperature in degrees Celsius. The follow sections present specific examples of this for several popular programming languages.
Terminal one-liner
Let’s start off with a terminal one-liner. Basically, this offers an alternative to the Raspbian vcgencmd measure_temp
command.
head -n 1 /sys/class/thermal/thermal_zone0/temp | xargs -I{} awk "BEGIN {printf \"%.2f\n\", {}/1000}"
The first line in file /sys/class/thermal/thermal_zone0/temp
is read and then piped to awk
with the help of xargs
. awk
converts the CPU temperature to a floating point value in degrees Celsius.
Python
The following Python script contains a reusable function get_cpu_temp()
that you can copy to your own Python program. Additionally, it contains a test program implemented in function main()
to demonstrate how to use the get_cpu_temp()
function.
#!/usr/bin/env python3 import os def main(): """ Program to demonstrate how to obtain the current value of the CPU temperature. """ print('Current CPU temperature is {:.2f} degrees Celsius.'.format(get_cpu_temp())) def get_cpu_temp(): """ Obtains the current value of the CPU temperature. :returns: Current value of the CPU temperature if successful, zero value otherwise. :rtype: float """ # Initialize the result. result = 0.0 # The first line in this file holds the CPU temperature as an integer times 1000. # Read the first line and remove the newline character at the end of the string. if os.path.isfile('/sys/class/thermal/thermal_zone0/temp'): with open('/sys/class/thermal/thermal_zone0/temp') as f: line = f.readline().strip() # Test if the string is an integer as expected. if line.isdigit(): # Convert the string with the CPU temperature to a float in degrees Celsius. result = float(line) / 1000 # Give the result back to the caller. return result if __name__ == "__main__": main()
To use this script, save it as cputemp.py
. Next, make sure that the script is executable before running it:
chmod +x cputemp.py
./cputemp.py
Shell
The following shell script contains a reusable function get_cpu_temp()
that you can copy to your own shell script. Additionally, it contains a test program to demonstrate how to use the get_cpu_temp()
function.
#!/bin/bash #---------------------------------------------------------------------------------------- # Obtains the current value of the CPU temperature. #---------------------------------------------------------------------------------------- function get_cpu_temp() { # Initialize the result. local result=0.00 # The first line in this file holds the CPU temperature as an integer times 1000. # Read the first line from the file. line=$(head -n 1 /sys/class/thermal/thermal_zone0/temp) # Test if the string is an integer as expected with a regular expression. if [[ $line =~ ^-?[0-9]+$ ]] then # Convert the CPU temperature to degrees Celsius and store as a string. result=$(awk "BEGIN {printf \"%.2f\n\", $line/1000}") fi # Give the result back to the caller. echo "$result" } #---------------------------------------------------------------------------------------- # Program to demonstrate how to obtain the current value of the CPU temperature. #---------------------------------------------------------------------------------------- cputemp=$(get_cpu_temp) echo Current CPU temperature is $cputemp degrees Celsius.
To use this script, save it as cputemp.sh
. Next, make sure that the script is executable before running it:
chmod +x cputemp.sh
./cputemp.sh
C
The following C program contains a reusable function get_cpu_temp()
that you can copy to your own C program. Additionally, it contains a test program implemented in function main()
to demonstrate how to use the get_cpu_temp()
function.
/**************************************************************************************** * Include files ****************************************************************************************/ #include <stdio.h> #include <stdlib.h> #include <string.h> #include <ctype.h> /**************************************************************************************** * Function prototypes ****************************************************************************************/ float get_cpu_temp(void); /************************************************************************************//** ** \brief Program to demonstrate how to obtain the current value of the CPU ** temperature. ** \details You can build this program with the command: ** gcc cputemp.c -o cputemp ** \return Program exit code. ** ****************************************************************************************/ int main(void) { printf("Current CPU temperature is %.2f degrees Celsius\n", get_cpu_temp()); return 0; } /*** end of main ***/ /************************************************************************************//** ** \brief Obtains the current value of the CPU temperature. ** \return Current value of the CPU temperature if successful, zero value otherwise. ** ****************************************************************************************/ float get_cpu_temp(void) { float result; FILE * f; char line[256] = "0"; char * pos; /* Initialize the result. */ result = 0.0f; /* Open the file for reading. */ f = fopen("/sys/class/thermal/thermal_zone0/temp", "r"); /* Only continue if the file could be opened. */ if (f != NULL) { /* The first line in this file holds the CPU temperature as an integer times 1000. * Read the first line and remove the newline character at the end of the string. */ fgets(line, sizeof(line)/sizeof(line[0]), f); /* Close the file now that we are done with it. */ fclose(f); } /* The read line should now contain digits followed by a newline character. * Make it a string by locating the newline character and replacing it with * a string termination character. */ pos = strchr(line, '\n'); /* Was the newline character found? */ if (pos != NULL) { /* Replace it with a string termination character. */ *pos = 0; } /* No newline character found so something is wrong with the string. */ else { /* Reset the line to a safe dummy value. */ strcpy(line, "0"); } /* Verify that the line now contains just digits. */ pos = line; while (*pos != 0) { if (!isdigit(*pos)) { /* Reset the line to a safe dummy value. */ strcpy(line, "0"); break; } pos++; } /* Convert the line string to an integer, divide by zero while converting * to a float. */ result = ((float)atoi(line)) / 1000; /* Give the result back to the caller. */ return result; } /*** end of get_cpu_temp ***/
To use this program, save it as cputemp.c
. Next, compile the source-file and link it to an executable. Afterwards, you can directly run it:
gcc cputemp.c -o cputemp
./cputemp
Pascal
The following Pascal program contains a reusable function get_cpu_temp()
that you can copy to your own Pascal program. Additionally, it contains a test program to demonstrate how to use the get_cpu_temp()
function.
program cputemp; {$mode objfpc}{$H+} //*************************************************************************************** // Includes //*************************************************************************************** uses SysUtils; //*************************************************************************************** // NAME: get_cpu_temp // RETURN VALUE: Current value of the CPU temperature if successful, zero value // otherwise. // DESCRIPTION: Obtains the current value of the CPU temperature. // //*************************************************************************************** function get_cpu_temp: real; var f: text; line: string; begin // Initialize the result. result := 0.0; // Initialize line to a safe dummy value. line := '0'; // Only continue if the file exists. if FileExists('/sys/class/thermal/thermal_zone0/temp') then begin // Open the file for reading. Assign(f, '/sys/class/thermal/thermal_zone0/temp'); Reset(f); // Read the first line from the file and then close it again. readln(f, line); Close(f); end; // Convert the string with the CPU temperature to a float in degrees Celsius. try result := StrToInt(line) / 1000; except result := 0.0; end; end; //*** end of get_cpu_temp *** //*************************************************************************************** // NAME: main // RETURN VALUE: Current value of the CPU temperature if successful, zero value // otherwise. // DESCRIPTION: Program to demonstrate how to obtain the current value of the CPU // temperature. You can build this program with the command: // fpc cputemp.pas -ocputemp // //*************************************************************************************** begin writeln('Current CPU temperature is ' + FormatFloat('0.00', get_cpu_temp) + ' degrees Celsius.'); ExitCode := 0; end. //*** end of main ***/
To use this program, save it as cputemp.pas
. Next, compile the source-file and link it to an executable. Afterwards, you can directly run it:
fpc cputemp.pas -ocputemp
./cputemp
Wrap up
This article explained in detail how you can check the Raspberry PI CPU temperature. Code snippets for multiple programming languages were presented for you. You can integrate these code snippets into your own software program. For example to develop your own Raspberry PI CPU temperature monitor or logger program. Moreover, the implementation of these code snippets is such that they also work on other Linux distributions besides Raspbian. Note that you can download all presented code examples directly from PragmaticLinux’s CpuTemp GitHub repository.
you can do it in shell pretty much as easily as your example without needing to use awk:
#!/bin/bash
temp=`cat /sys/class/thermal/thermal_zone0/temp`
((foo = temp / 1000))
((bar = temp % 1000))
printf “%s temp=%d.%.3d\302\260C\n” `date +%x-%T` $foo $bar
That is a nice solution too. Thank you for sharing.