Looking for a way to create and array of strings in Python? You came to the right place. This hands-on article explains several ways of how to create an array of strings in Python. It includes example code snippets to demonstrate how to loop over the array elements as well.
Background
A few weeks ago I was helping someone write a Python script to automate their work-flow. At one point we needed to create a string array. Since it was a while since I last coded in Python, I didn’t have the syntax memorized on how to create an array of strings. What to do? A quick web search with your search engine of choice of course. Unfortunately, the top search hits did not yield the results we were looking for. Hence this article; a quick reference on how to create an array of strings in Python. Handy for myself and hopefully for others as well.
What do you need
To follow along with the presented examples, you just need a PC with the Python interpreter installed. Since the Python interpreter runs on pretty much all operating systems, you can use Windows, macOS or Linux throughout this article. Because this blog primarily focuses on Linux, that’s what I’ll use. My trusty openSUSE Tumbleweed machine to be exact. Curious about trying out openSUSE Tumbleweed? This article gets you started:
All popular Linux distributions come with Python installed. So theoretically, you just need a text editor for writing your Python script. I personally prefer a bit of a beefier development environment: the PyCharm IDE from JetBrains. They offer a community edition, which you can use free or charge. To install the PyCharm Community edition, I recommend its Flatpak edition as explained in this article:
We’ll need a Python script for testing out the presented code snippets. Here’s one to get you started. Save it in a file called stringarrays.py
:
#!/usr/bin/env python3 def main(): # TODO Enter code here.. pass if __name__ == "__main__": main()
To try out a code snippet, replace the pass
line with the code snippet and then run your Python script.
Arrays in Python
First things first: What is an array? The following list sums it up:
- An array is a list of variables of the same data type.
- Array elements are accessed with a zero-based index.
- You can dynamically add, remove and swap array elements.
If you know your way around a spreadsheet, you can think of an array as a one-column spreadsheet. The name of the column is the name of the array itself. Likewise, the row number is the index of an array element. You just need to keep in mind that an array index starts at 0
and not at 1
.
Time for an example. Let’s say you have a bunch of numbers (1000
, 1500
, 1877
and 496
) that you want to store in an array. One way of declaring and initializing an array variable that holds these values:
# Create an array with 4 numbers my_numbers = [1000, 1500, 1877, 496]
Alternatively, you can construct the array dynamically:
# Create an empty array my_numbers = [] # Add array elements one at a time my_numbers.append(1000) my_numbers.append(1500) my_numbers.append(1877) my_numbers.append(496)
To access the element at a specific index in the array, you use square brackets. Example:
# Read the value at index 1 my_value = my_numbers[1] # Print the value print(my_value)
This prints out the value 1500
.

Strings in Python
A string in Python is basically a variable that stores text. You surround your text by either single quotes or double quotes. Which one doesn’t really matter. It’s just a way of telling Python that the value is text and not numerical. For example:
# Create and initialize a string variable my_string = 'Just some random text.' # Print the value print(my_string)

Since we already covered arrays in the previous section, you can also understand strings like this: A string is nothing more than an array of characters. So each array element holds a character of the string. To print the 4th character of the previous string:
# Read the character at index 3 my_value = my_string[3] # Print the character print(my_value)
This prints out the character t
. So the last letter of the word Just
that we stored in string variable my_string
.

Create an array of strings in Python
Now that we know about strings and arrays in Python, we simply combine both concepts to create and array of strings. For example to store different pets. To declare and initialize an array of strings in Python, you could use:
# Create an array with pets my_pets = ['Dog', 'Cat', 'Bunny', 'Fish']
Just like we saw before, you can also construct the array one element at a time:
# Create an empty array my_pets = [] # Add array elements one at a time my_pets.append('Dog') my_pets.append('Cat') my_pets.append('Bunny') my_pets.append('Fish')
Since it’s an array, you can access each element using square brackets and specifying its zero based index. To read and print the 3rd pet in the string array, we use index 2
:
# Read the value at index 2 my_pet = my_pets[2] # Print the value print(my_pet)
This prints out Bunny
:

In case you don’t know which pet to get in real life, you could let Python decide for you, using its random number generator. To access the random number generator, import its module by adding this line at the top of your Python file:
import random
We can now generate a random number between 0 and 3, which later serves as an index into our string array with pets:
my_pet_index = random.randint(0, 3)
Putting it all together to request Python to decide on a pet for us:
my_pets = ['Dog', 'Cat', 'Bunny', 'Fish'] my_pet_index = random.randint(0, 3) my_pet = my_pets[my_pet_index] print(my_pet)
In my case, it turns out Python wants me to get a Bunny
:

Loop over a string array in Python
When storing strings (or other data) in an array, you do so for a purpose in your Python program. Working with the resulting array, typically involves looping over its contents. This looping is sometimes called iterating. Several methods exists for looping over an array. The most concise one:
for pet in my_pets: print(pet)
These two lines of code pack a whole lot of functionality:
- It loops over each array element in string array
my_pets
. - The current element is stored in a variable called
pet
. Note that you can change this variable name to whatever you prefer. - Inside the loop, we print out the name of the current element.
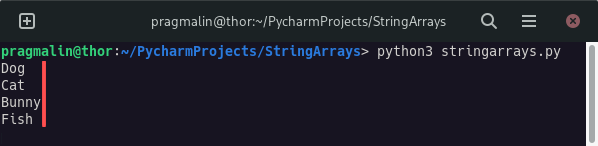
A more verbose approach with an array indexer:
pet_index = 0 while pet_index < len(my_pets): print(my_pets[pet_index]) pet_index += 1
- The first line declares and initializes an indexer variable
pet_index
. You can change this variable name to something else, if your prefer. - The loop repeats as long as the
pet_index
value is less than the total number of elements in themy_pets
array. - Inside the loop body, we:
- Print out the name of the array element at the current index.
- Increment the
pet_index
value by one in preparation for the next loop iteration.
Both methods for looping over an array in Python do the same. Whichever method you use is up to your own personal preference.
Wrap up
This article set out to explain how to create an array of strings in Python. Before diving into this topic, we first learned how about arrays and strings, independently. Afterwards, we combined what we learned and applied it to create string arrays. Afterwards, we covered looping through array elements. As an often used technique, this article would haven been incomplete without covering it.